Java Weather Application With Code And Explanation
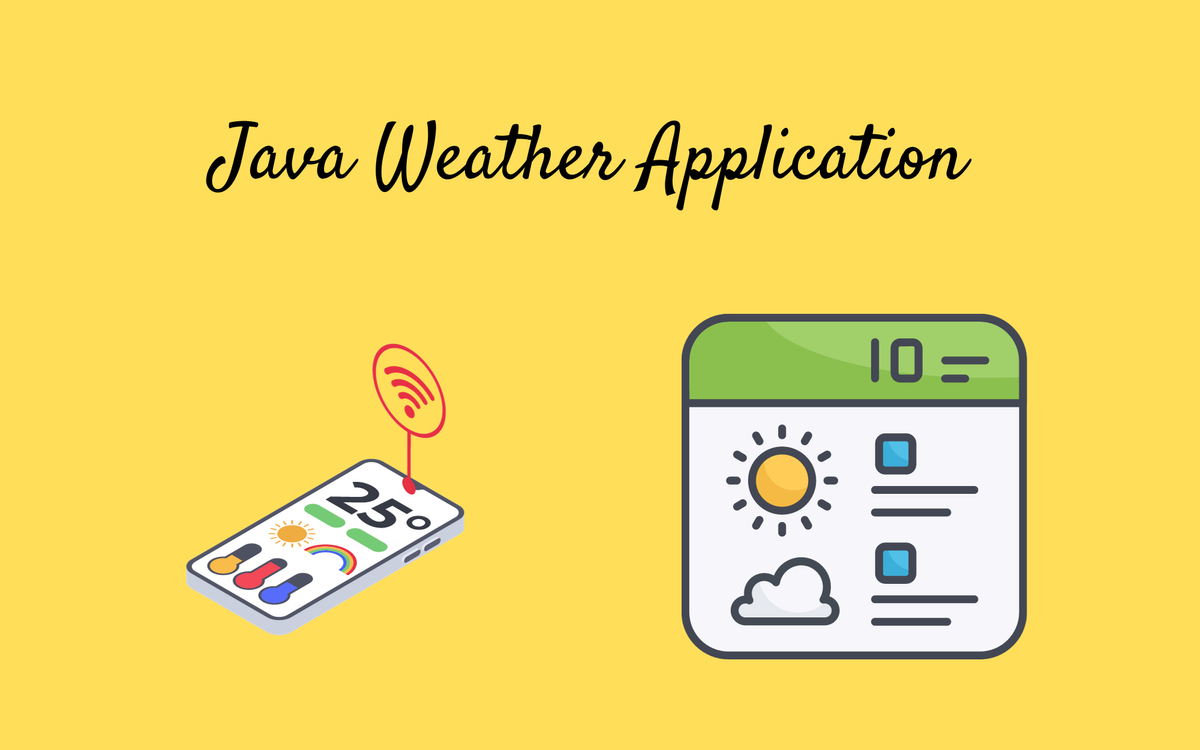
This project will fetch weather data for a specific city and display it in the console.
Step 1: Set Up Your Environment
Before you start coding, make sure you have Java installed on your computer.
Step 2: Get an API Key
You'll need an API key from OpenWeatherMap. You can sign up on their website to get a free API key.
Step 3: Create a Java Class
Create a new Java class (e.g., WeatherApp.java
) and open it in your favorite code editor.
Step 4: Write the Java Code
Here's the Java code for the weather project:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class WeatherApp {
// Replace with your own API key from OpenWeatherMap
private static final String API_KEY = "YOUR_API_KEY";
public static void main(String[] args) {
String city = "London"; // Replace with the desired city name
try {
String weatherData = getWeatherData(city);
System.out.println(weatherData);
} catch (Exception e) {
e.printStackTrace();
}
}
public static String getWeatherData(String city) throws Exception {
String apiUrl = "http://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=" + API_KEY;
URL url = new URL(apiUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder result = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
result.append(line);
}
reader.close();
return result.toString();
}
}
Explanation:
- Import necessary packages:
java.io.BufferedReader
andjava.io.InputStreamReader
for reading data from the API response.java.net.HttpURLConnection
andjava.net.URL
for making HTTP requests.
- Replace
YOUR_API_KEY
with your actual API key from OpenWeatherMap. - In the
main
method, specify the city for which you want to get weather data. - The
getWeatherData
method takes a city name as input, constructs the API URL, and makes a request to OpenWeatherMap's API to get weather data for that city. - The method then reads the response and returns it as a string.
Step 5: Run the Code
Compile and run the Java file. You should see the weather data for the specified city printed in the console.
Keep in mind that this is a simple console-based project. For a more advanced weather app, you might want to consider building a graphical user interface (GUI) or a web-based application. Additionally, you could add error handling, user input validation, and more features to enhance the project.