React Hook: A Guide To Beginners
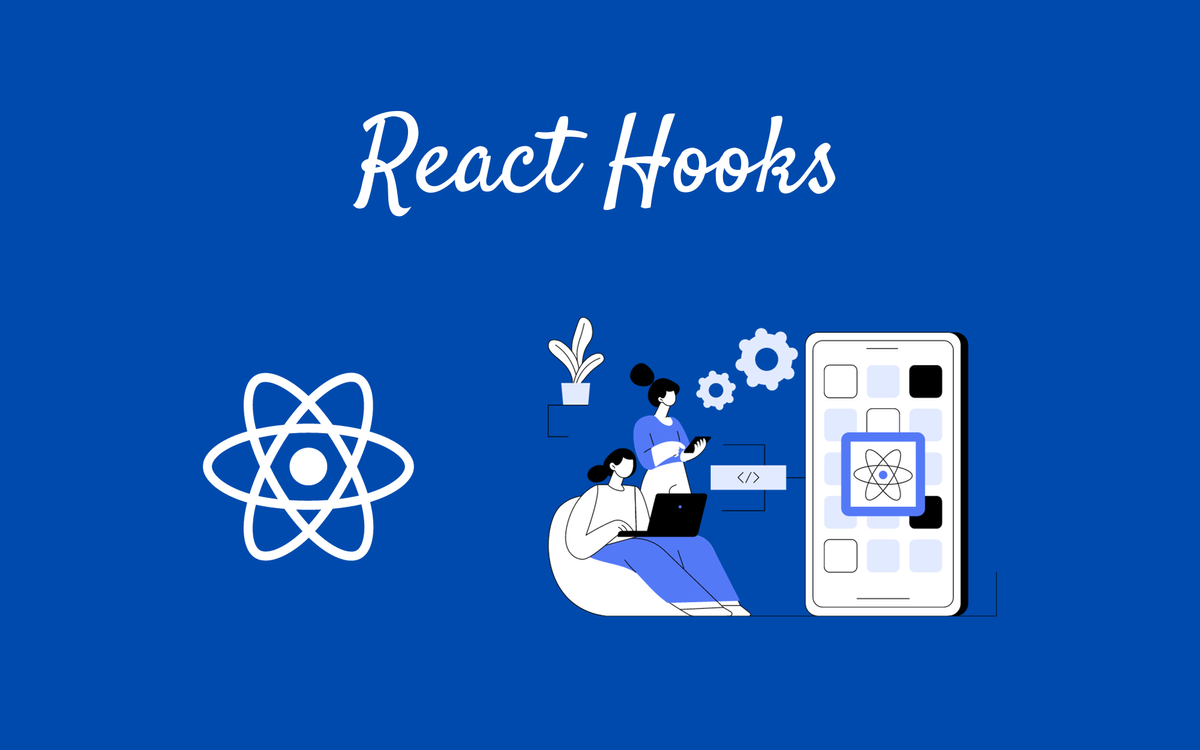
React Hooks were introduced in React 16.8 to allow functional components to manage state and side effects. They provide a way to use stateful logic without writing a class component. Here are some commonly used hooks with examples and explanations:
1. useState
The useState
hook allows functional components to manage local state.
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
export default Counter;
useState
returns an array with two elements: the current state value (count
) and a function (setCount
) to update it.setCount
can take a new value or a function that receives the current state and returns a new state.
2. useEffect
The useEffect
hook allows functional components to perform side effects.
import React, { useState, useEffect } from 'react';
const ExampleComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default ExampleComponent;
useEffect
accepts a function that contains the code for the side effect.- The second argument is an array of dependencies. If any of the dependencies change, the effect will be re-run.
3. useContext
The useContext
hook allows functional components to consume context.
import React, { useContext } from 'react';
const MyComponent = () => {
const value = useContext(MyContext);
return <div>{value}</div>;
};
useContext
takes a context object and returns the current context value.
4. useReducer
The useReducer
hook is a more powerful alternative to useState
.
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</>
);
}
useReducer
takes a reducer function and an initial state. It returns the current state and a dispatch function to update it.
5. useMemo and useCallback
These hooks are used for performance optimization.
import React, { useMemo, useCallback } from 'react';
const MemoizedComponent = ({ items }) => {
const total = useMemo(() => items.reduce((acc, item) => acc + item, 0), [items]);
const handleClick = useCallback(() => {
console.log('Button clicked!');
}, []);
return (
<div>
<p>Total: {total}</p>
<button onClick={handleClick}>Click Me</button>
</div>
);
};
These hooks help prevent unnecessary re-rendering of components.
These are some of the commonly used hooks in React. They enable functional components to manage state, perform side effects, and interact with context and reducers. Understanding and utilizing hooks effectively can lead to cleaner and more maintainable React code.